인스턴스 만들기
https://www.youtube.com/watch?v=rdlHszMujnw&list=PLfth0bK2MgIan-SzGpHIbfnCnjj583K2m&index=8
자세한 설명
https://www.youtube.com/watch?v=N8TB_6AbaM4&list=PLfth0bK2MgIan-SzGpHIbfnCnjj583K2m&index=11
이론 공부는 이 정도로 마무리하고, 이제 내 코드를 직접 올린 서버를 만들고 싶어졌습니다!
지금 제 필을 올리고는 싶지만, 필은 이것저것 많이 되어있기 때문에 무섭습니다!
새로 코드를 한장 작성했습니다.
using System.Net;
using System.Net.Sockets;
using JsonSerializer = System.Text.Json.JsonSerializer;
public class TcpTest
{
public static async Task Main(string[] args)
{
IPEndPoint endPoint = new IPEndPoint(IPAddress.Any, 51225);
Socket serverSocket = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
serverSocket.Bind(endPoint);
serverSocket.Listen(1);
Socket clientSocket = await serverSocket.AcceptAsync();
byte[] receiveBuffer = new byte[1024];
byte[] sendBuffer = new byte[1024];
int length = await clientSocket.ReceiveAsync(receiveBuffer, SocketFlags.None);
string rcv = System.Text.Encoding.UTF8.GetString(receiveBuffer, 0, length);
if (rcv == "" || rcv.Length == 0)
return;
if (clientSocket.Connected == false)
return;
var response = rcv + " Did you send this ~?";
Console.WriteLine(response);
string json = JsonSerializer.Serialize(response);
sendBuffer = System.Text.Encoding.UTF8.GetBytes(json);
clientSocket.Send(sendBuffer, 0, json.Length, SocketFlags.None);
clientSocket.Close();
}
}
이 코드를 깃허브에 올렸습니다.
https://github.com/MatorMirne/TcpTestServer
받은 메세지를 출력하고 꺼지는 간단한 프로그램입니다.
클라이언트 코드는 아래와 같습니다.
using System.Net;
using System.Net.Sockets;
using System.Text.Json;
IPAddress ipAddress = IPAddress.Parse("127.0.0.1");
IPEndPoint endPoint = new IPEndPoint(ipAddress, 51225);
Socket socket;
socket = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
socket.Connect(endPoint);
byte[] receiveBuffer = new byte[1024];
byte[] sendBuffer = new byte[1024];
var input = Console.ReadLine();
Console.WriteLine($"request:{input}");
sendBuffer = System.Text.Encoding.UTF8.GetBytes(input);
await Task.Run( () => socket.SendAsync(sendBuffer));
int length = 0;
try
{
length = await socket.ReceiveAsync(receiveBuffer);
}
catch (SocketException e)
{
if (e.ErrorCode == 89) ; // 듣기 중단 (들을거 없음)
if (e.ErrorCode == 57) ; // 듣기 중단 (연결 종료)
else throw;
}
catch (ObjectDisposedException e)
{
if (e.ObjectName == "System.Net.Sockets.Socket") ; // 소켓 반납 이후에는 듣지 않음
else throw;
}
string receiveMessage = System.Text.Encoding.UTF8.GetString(receiveBuffer, 0, length);
Console.WriteLine($"수신 : {receiveMessage}");
이렇게 하면 통신을 할 수 있을것같아요 !
그럼 EC2 인스턴스를 하나 만들어 보겠습니다!
저는 51225번 포트를 사용하므로, 개방해 주었습니다.
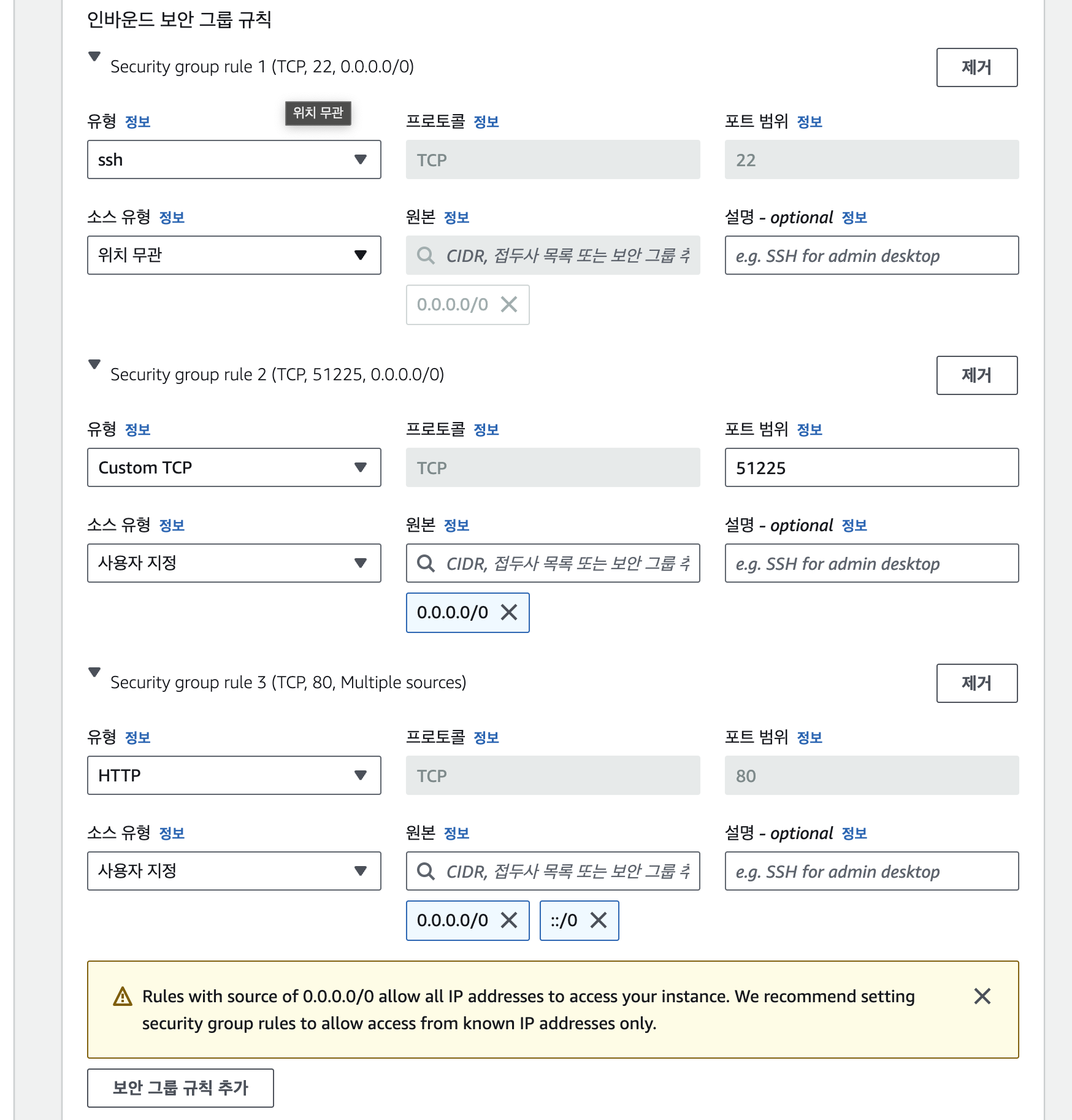
이제 Git 에서 코드를 내려받고 싶은데요 ...
아래 글을 보고 따라하는데, 진짜 너무너무 무섭습니다 ㅋㅋ ㅠㅠㅠㅠ
git 을 설치하는 과정을 따라하는데, 과금이 너무 두려워서 일시 중단했습니다.
내일 예산 리포트 받아보고 마저 진행하도록 하겠습니다.
Git 설치 (Source)
이번에는 Source를 이용한 Git 설치를 알아보겠습니다. Git 사이트로 부터 파일을 내려받기 위해 wget을 설치합니다. yum install wget wget 설치가 완료되었습니다. git 설치에 앞서 다운로드 받을 Git Source
bbada.tistory.com
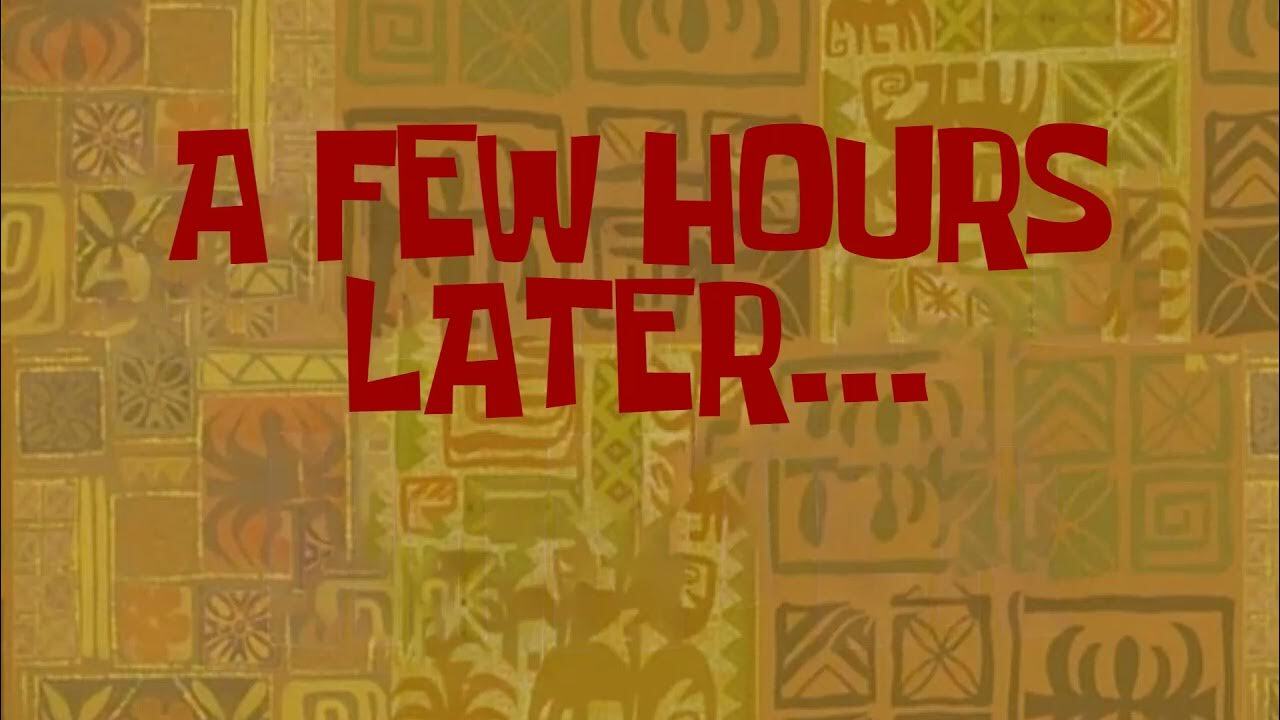
오 !!! 과금이 전혀 되지 않았습니다 ㅎㅎ 만세
그럼 본격적으로 원격 가상머신에 제 코드를 심어 보겠습니다 ㅎㅎ
우선 제가 51225번 포트를 쓴다는 사실이 널리 알려졌으므로(?) 포트 번호를 변경하겠습니다.

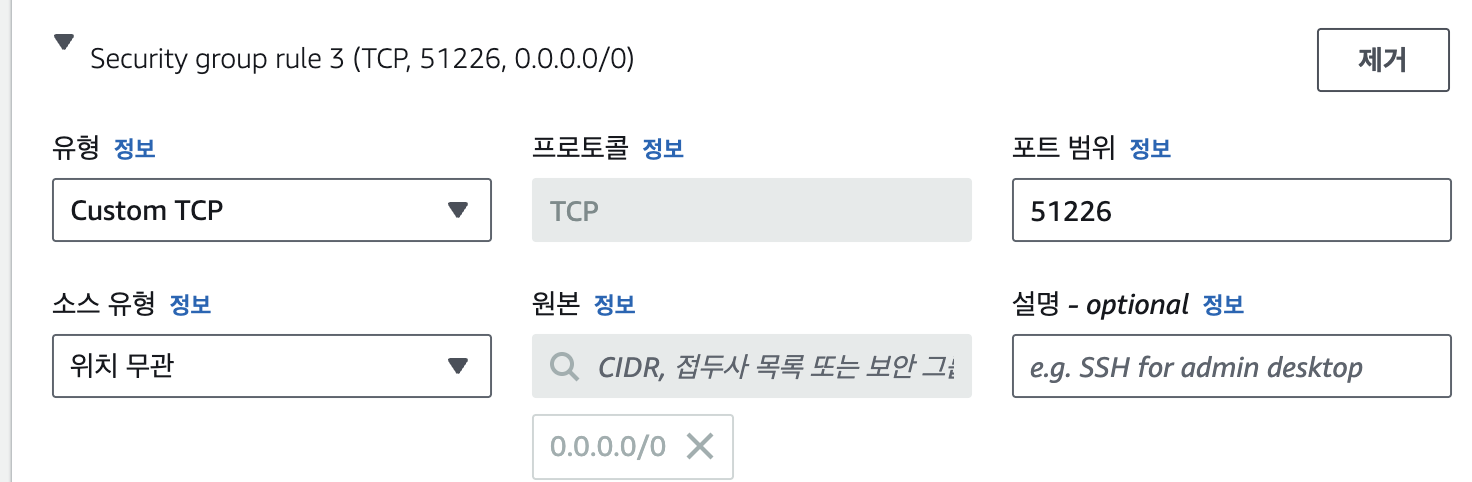
위 빈칸이 제일 헷갈리네요.
Tcp 51226 번 포트를 허용하기 위해서는 이렇게 하면 되겠죠 ?
인스턴스를 시작하고 연결해 주었습니다.
깃을 설치하고 저장소를 복제합니다. 그리고 원격 환경에서 프로젝트를 실행시키고
// git 설치
sudo yum install git
// git clone
git clone https://github.com/MatorMirne/TcpTestServer.git
// dotnet 설치
sudo yum install dotnet
// dotnet 버전 업그레이드
sudo dnf install dotnet-sdk-7.0 --allowerasing
// dotnet 버전 확인
dotnet --version
// 위치로 이동하여, 서버 실행
cd TcpTestServer/bin/Debug/net7.0
dotnet TcpTestServer.dll
제 컴퓨터에서 Tcp 신호를 보냈는데 잘 받습니다.

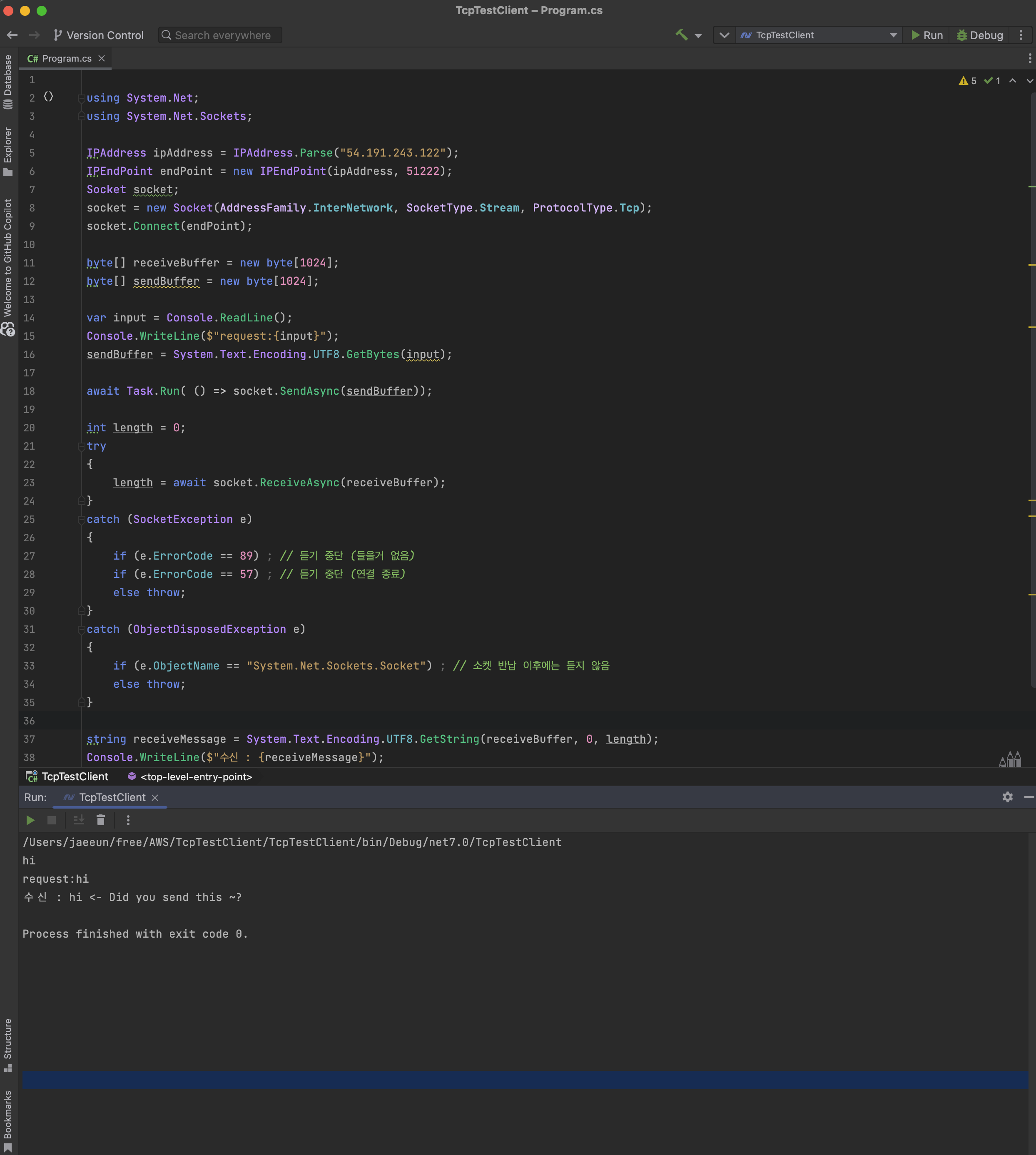
실습 끝
'일지' 카테고리의 다른 글
AWS 공부일지 : 이론 (1) | 2023.11.09 |
---|---|
iTerm2 여러 창 자동으로 매크로(?) 세팅하는 법 설명 / iTerm Script 예시 (0) | 2023.08.07 |
인스턴스 만들기
https://www.youtube.com/watch?v=rdlHszMujnw&list=PLfth0bK2MgIan-SzGpHIbfnCnjj583K2m&index=8
자세한 설명
https://www.youtube.com/watch?v=N8TB_6AbaM4&list=PLfth0bK2MgIan-SzGpHIbfnCnjj583K2m&index=11
이론 공부는 이 정도로 마무리하고, 이제 내 코드를 직접 올린 서버를 만들고 싶어졌습니다!
지금 제 필을 올리고는 싶지만, 필은 이것저것 많이 되어있기 때문에 무섭습니다!
새로 코드를 한장 작성했습니다.
using System.Net;
using System.Net.Sockets;
using JsonSerializer = System.Text.Json.JsonSerializer;
public class TcpTest
{
public static async Task Main(string[] args)
{
IPEndPoint endPoint = new IPEndPoint(IPAddress.Any, 51225);
Socket serverSocket = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
serverSocket.Bind(endPoint);
serverSocket.Listen(1);
Socket clientSocket = await serverSocket.AcceptAsync();
byte[] receiveBuffer = new byte[1024];
byte[] sendBuffer = new byte[1024];
int length = await clientSocket.ReceiveAsync(receiveBuffer, SocketFlags.None);
string rcv = System.Text.Encoding.UTF8.GetString(receiveBuffer, 0, length);
if (rcv == "" || rcv.Length == 0)
return;
if (clientSocket.Connected == false)
return;
var response = rcv + " Did you send this ~?";
Console.WriteLine(response);
string json = JsonSerializer.Serialize(response);
sendBuffer = System.Text.Encoding.UTF8.GetBytes(json);
clientSocket.Send(sendBuffer, 0, json.Length, SocketFlags.None);
clientSocket.Close();
}
}
이 코드를 깃허브에 올렸습니다.
https://github.com/MatorMirne/TcpTestServer
받은 메세지를 출력하고 꺼지는 간단한 프로그램입니다.
클라이언트 코드는 아래와 같습니다.
using System.Net;
using System.Net.Sockets;
using System.Text.Json;
IPAddress ipAddress = IPAddress.Parse("127.0.0.1");
IPEndPoint endPoint = new IPEndPoint(ipAddress, 51225);
Socket socket;
socket = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
socket.Connect(endPoint);
byte[] receiveBuffer = new byte[1024];
byte[] sendBuffer = new byte[1024];
var input = Console.ReadLine();
Console.WriteLine($"request:{input}");
sendBuffer = System.Text.Encoding.UTF8.GetBytes(input);
await Task.Run( () => socket.SendAsync(sendBuffer));
int length = 0;
try
{
length = await socket.ReceiveAsync(receiveBuffer);
}
catch (SocketException e)
{
if (e.ErrorCode == 89) ; // 듣기 중단 (들을거 없음)
if (e.ErrorCode == 57) ; // 듣기 중단 (연결 종료)
else throw;
}
catch (ObjectDisposedException e)
{
if (e.ObjectName == "System.Net.Sockets.Socket") ; // 소켓 반납 이후에는 듣지 않음
else throw;
}
string receiveMessage = System.Text.Encoding.UTF8.GetString(receiveBuffer, 0, length);
Console.WriteLine($"수신 : {receiveMessage}");
이렇게 하면 통신을 할 수 있을것같아요 !
그럼 EC2 인스턴스를 하나 만들어 보겠습니다!
저는 51225번 포트를 사용하므로, 개방해 주었습니다.
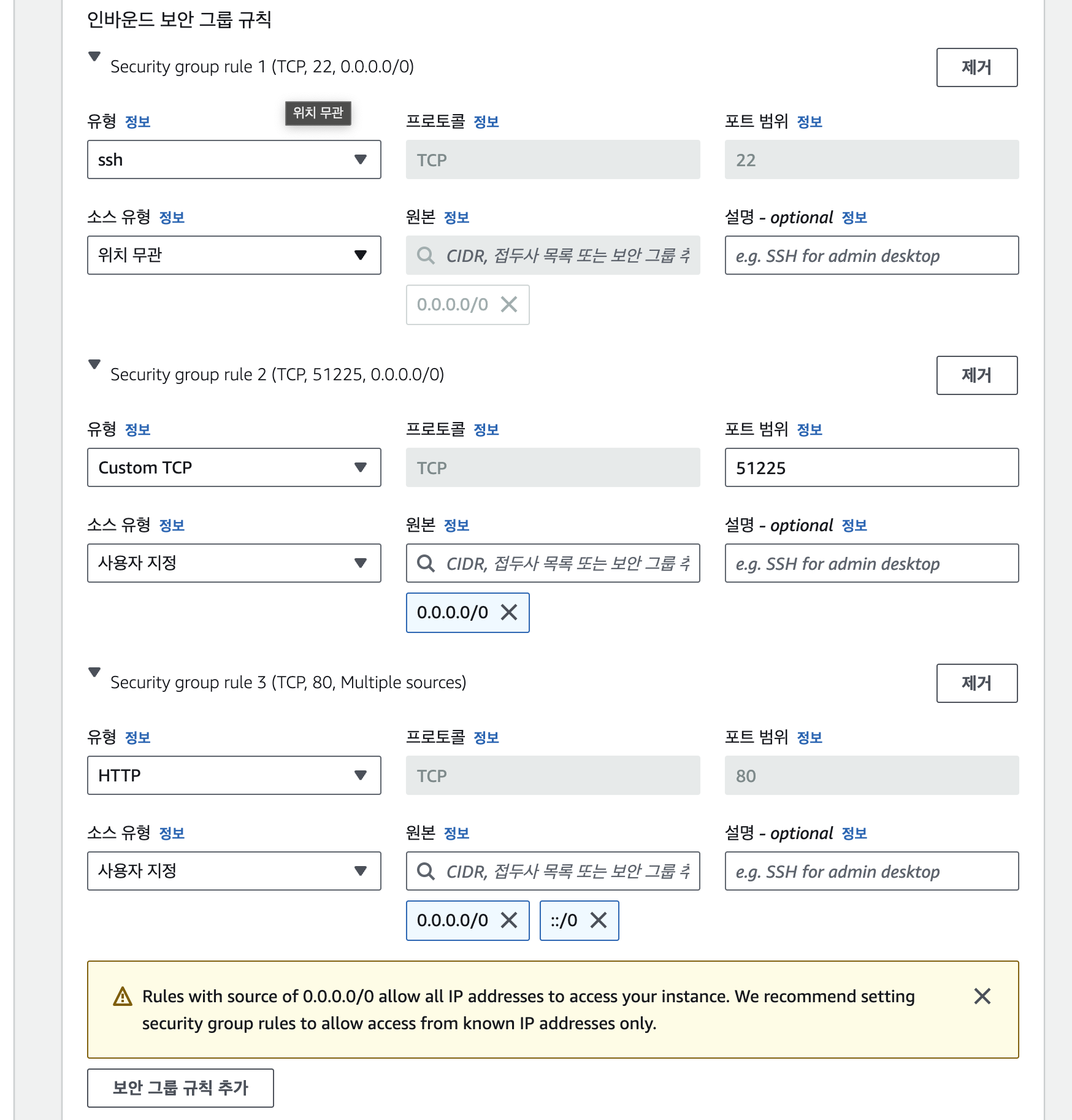
이제 Git 에서 코드를 내려받고 싶은데요 ...
아래 글을 보고 따라하는데, 진짜 너무너무 무섭습니다 ㅋㅋ ㅠㅠㅠㅠ
git 을 설치하는 과정을 따라하는데, 과금이 너무 두려워서 일시 중단했습니다.
내일 예산 리포트 받아보고 마저 진행하도록 하겠습니다.
Git 설치 (Source)
이번에는 Source를 이용한 Git 설치를 알아보겠습니다. Git 사이트로 부터 파일을 내려받기 위해 wget을 설치합니다. yum install wget wget 설치가 완료되었습니다. git 설치에 앞서 다운로드 받을 Git Source
bbada.tistory.com
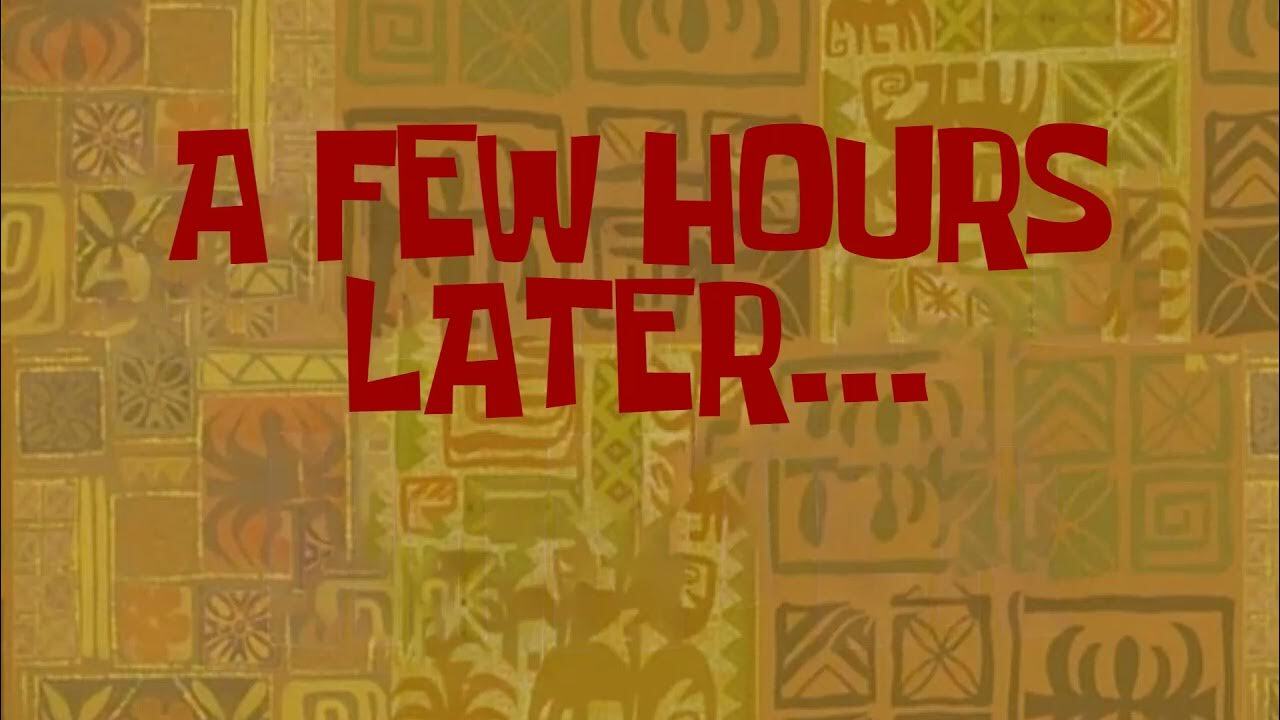
오 !!! 과금이 전혀 되지 않았습니다 ㅎㅎ 만세
그럼 본격적으로 원격 가상머신에 제 코드를 심어 보겠습니다 ㅎㅎ
우선 제가 51225번 포트를 쓴다는 사실이 널리 알려졌으므로(?) 포트 번호를 변경하겠습니다.

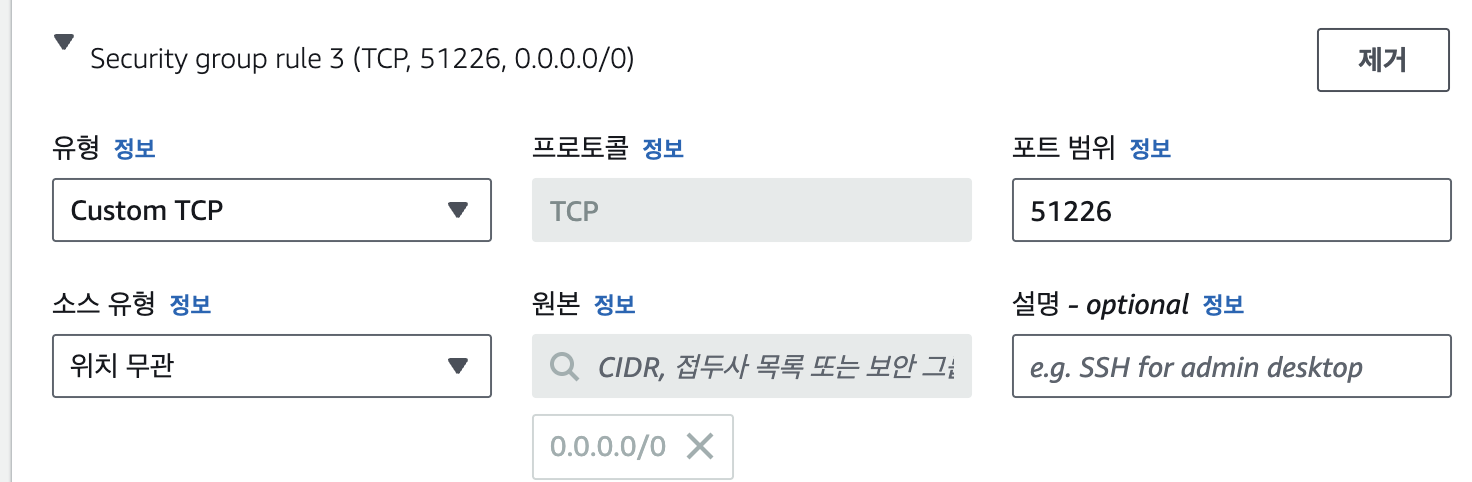
위 빈칸이 제일 헷갈리네요.
Tcp 51226 번 포트를 허용하기 위해서는 이렇게 하면 되겠죠 ?
인스턴스를 시작하고 연결해 주었습니다.
깃을 설치하고 저장소를 복제합니다. 그리고 원격 환경에서 프로젝트를 실행시키고
// git 설치
sudo yum install git
// git clone
git clone https://github.com/MatorMirne/TcpTestServer.git
// dotnet 설치
sudo yum install dotnet
// dotnet 버전 업그레이드
sudo dnf install dotnet-sdk-7.0 --allowerasing
// dotnet 버전 확인
dotnet --version
// 위치로 이동하여, 서버 실행
cd TcpTestServer/bin/Debug/net7.0
dotnet TcpTestServer.dll
제 컴퓨터에서 Tcp 신호를 보냈는데 잘 받습니다.

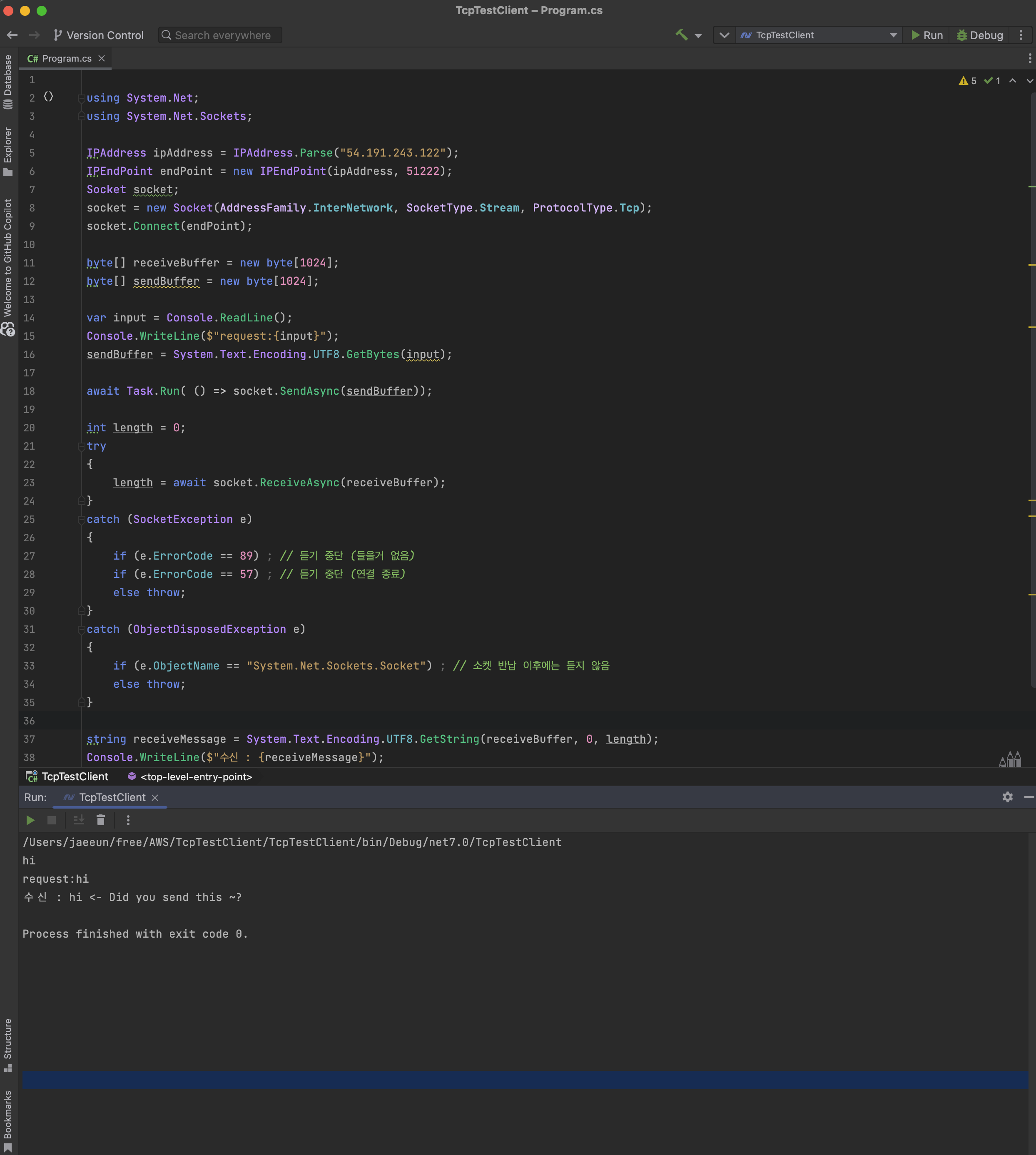
실습 끝
'일지' 카테고리의 다른 글
AWS 공부일지 : 이론 (1) | 2023.11.09 |
---|---|
iTerm2 여러 창 자동으로 매크로(?) 세팅하는 법 설명 / iTerm Script 예시 (0) | 2023.08.07 |